Performance Tips for Android's ListView
April 05, 2012
I’ve been messing around with Android-based code for a few months now while hacking on Native Firefox for Android and Pattrn. I noticed that the performance tips for ListViews are a bit scattered in different sources. This post is an attempt to summarize the ones I found most useful.
I’m assuming you’re already familiar with ListViews and understand the framework around AdapterViews. I’ve added some Android source code pointers for the curious readers willing to understand things a bit deeper.
How it works
ListView is designed for scalability and performance. In practice, this essentially means:
- It tries to do as few view inflations as possible.
- It only paints and lays out children that are (or are about to become) visible on screencode.
The reason for 1 is simple: layout inflations are expensive operationscode. Although layout files are compiled into binary form for more efficient parsingcode, inflations still involve going through a tree of special XML blockscode and instantiating all respective views. ListView solves this problem by recyclingcode non-visible views—called “ScrapViews” in Android’s source code—as you pan around. This means that developers can simply update the contents of recycled viewscode instead of inflating the layout of every single row—more on that later.
In order to implement 2, ListView uses the view recycler to keep adding recycled views below or above the current viewport and moving active views to a recyclable pool as they move off-screencode while scrolling. This way ListView only needs to keep enough views in memory to fill its allocated space in the layout and some additional recyclable views—even when your adapter has hundreds of items. It will fill the space with rows in different ways—from top, from bottom, etc—depending on how the viewport changedcode. The image below visually summarizes what happens when you pan a ListView down.
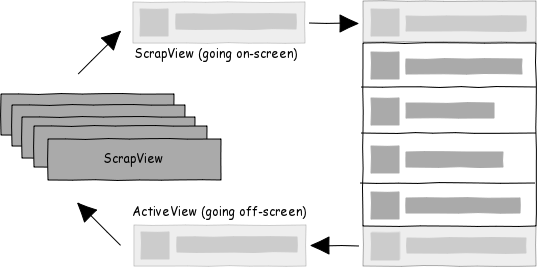
With this framework in mind, let’s move on to the tips. As you’ve seen above, ListView dynamically inflates and recycles tons of views when scrolling so it’s key to make your adapter’s getView() as lightweight as possible. All tips resolve around making getView() faster in one way or another.
View recycling
Every time ListView needs to show a new row on screen, it will call the getView() method from its adapter. As you know, getView() takes three arguments arguments: the row position, a convertView, and the parent ViewGroup.
The convertView argument is essentially a “ScrapView” as described earlier. It will have a non-null value when ListView is asking you recycle the row layout. So, when convertView is not null, you should simply update its contents instead of inflating a new row layout. The getView() code in your adapter would look a bit like:
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = mInflater.inflate(R.layout.your_layout, null);
}
TextView text = (TextView) convertView.findViewById(R.id.text);
text.setText("Position " + position);
return convertView;
}
View Holder pattern
Finding an inner view inside an inflated layout is among the most common operations in Android. This is usually done through a View method called findViewById(). This method will recursively go through the view tree looking for a child with a given IDcode. Using findViewById() on static UI layouts is totally fine but, as you’ve seen, ListView calls the adapter’s getView() very frequently when scrolling. findViewById() might perceivably hit scrolling performance in ListViews—especially if your row layout is non-trivial.
The View Holder pattern is about reducing the number of findViewById() calls in the adapter’s getView(). In practice, the View Holder is a lightweight inner class that holds direct references to all inner views from a row. You store it as a tag in the row’s view after inflating it. This way you’ll only have to use findViewById() when you first create the layout. Here’s the previous code sample with View Holder pattern applied:
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
convertView = mInflater.inflate(R.layout.your_layout, null);
holder = new ViewHolder();
holder.text = (TextView) convertView.findViewById(R.id.text);
convertView.setTag(holder);
} else {
holder = convertView.getTag();
}
holder.text.setText("Position " + position);
return convertView;
}
private static class ViewHolder {
public TextView text;
}
Async loading
Very often Android apps show richer content in each ListView row such as images. Using drawable resources in your adapter’s getView() is usually fine as Android caches those internallycode. But you might want to show more dynamic content—coming from local disk or internet—such as thumbnails, profile pictures, etc. In that case, you probably don’t want to load them directly in your adapter’s getView() because, well, you should never ever block UI thread with IO. Doing so means that scrolling your ListView would look anything but smooth.
What you want to do is running all per-row IO or any heavy CPU-bound routine asynchronously in a separate thread. The trick here is to do that and still comply with ListView’s recycling behaviour. For instance, if you run an AsyncTask to load a profile picture in the adapter’s getView(), the view you’re loading the image for might be recycled for another position before the AsyncTask finishes. So, you need a mechanism to know if the view hasn’t been recycled once you’re done with the async operation.
One simple way to achieve this is to attach some piece of information to the view that identifies which row is associated with it. Then you can check if the target row for the view is still the same when the async operation finishes. There are many ways of achieving this. Here is just a simplistic sketch of one way you could do it:
public View getView(int position, View convertView,
ViewGroup parent) {
ViewHolder holder;
...
holder.position = position;
new ThumbnailTask(position, holder)
.executeOnExecutor(AsyncTask.THREAD_POOL_EXECUTOR, null);
return convertView;
}
private static class ThumbnailTask extends AsyncTask {
private int mPosition;
private ViewHolder mHolder;
public ThumbnailTask(int position, ViewHolder holder) {
mPosition = position;
mHolder = holder;
}
@Override
protected Cursor doInBackground(Void... arg0) {
// Download bitmap here
}
@Override
protected void onPostExecute(Bitmap bitmap) {
if (mHolder.position == mPosition) {
mHolder.thumbnail.setImageBitmap(bitmap);
}
}
}
private static class ViewHolder {
public ImageView thumbnail;
public int position;
}
Interaction awareness
Asynchronously loading heavier assets for each row is an important step to towards a performant ListView. But if you blindly start an asynchronous operation on every getView() call while scrolling, you’d be wasting a lot of resources as most of the results would be discarded due to rows being recycled very often.
We need to add interaction awareness to your ListView adapter so that it doesn’t trigger any asynchronous operation per row after, say, a fling gesture on the ListView—which means that the scrolling is so fast that it doesn’t make sense to even start any asynchronous operation. Once scrolling stops, or is about to stop, is when you want to start actually showing the heavy content for each row.
I won’t post a code sample for this—as it involves too much code to post here—but the classic Shelves app by Romain Guy has a pretty good example. It basically triggers the async book cover loading once the GridView stops scrolling among other things. You can also balance interaction awareness with an in-memory cache so that you show cached content even while scrolling. You got the idea.
That’s all!
I strongly recommend watching Romain Guy and Adam Powell’s talk about ListView as it covers a lot of the stuff I wrote about here. Have a look at Pattrn if you want to see these tips in action. There’s nothing new about the tips in this post but I thought it would be useful to document them all in one place. Hopefully, it will be a useful reference for hackers getting started on Android development.
Update. I’ve just announced Smoothie, a tiny library that that offers an easy way to do async loading in ListViews/GridViews. It incorporates most of the tips described in this blog post behind a very simple API. Have a look!